|
CS 334
Programming Languages
Spring 2000
Lecture 1
|
Do programming languages matter?
Absolutely!!!
Otherwise, why would the Java folks have rejected C++ for their
programming language?
Explore in this course all aspects of programming languages, including
their features, type systems, programming styles supported, and
implementations.
Programming languages are the main interface between computers and
programmers, allowing us to express or understand algorithms to be
executed by the computer.
Abstractions
By providing abstractions (or mechanisms to create abstractions) they
influence the way we think about problems.
Data abstractions:
-
basic data types: integers, reals, booleans, characters, pointers
-
structured: arrays, records
-
unit: E.g., support for ADT's, modules, packages, classes
Control abstractions:
-
basic: assignment, goto, sequencing
-
structured: if..then..else.., loops, procedures & functions
-
unit: separately compiled units, modules, packages, concurrent tasks
Syntax and Semantics
For all constructs need to have clearly specified syntax and
semantics:
-
syntax: what is a legal expression?
-
semantics: what is the result when it is executed?
Syntax always given formally (as well as informally).
Semantics usually given informally (English), but more and more
formally.
Both necessary in order to ensure programs give predictable results.
How can programming languages support the software development
process?
Phases in the development process are:
-
Requirements
-
Specification
-
Implementation
-
Certification or Validation (includes testing and verification)
-
Maintenance
Need to evaluate languages with respect to overall picture. Not good
if just supports one aspect. Important to evaluate language based on what
its goals are.
-
BASIC - quick development of interactive programs
-
Pascal - instruction
-
C - low-level systems programming
-
FORTRAN - number crunching scientific computation
Languages which are good for quick hacking together of programs may not
be suitable for large-scale software development.
Better choices for large-scale software include:
-
Ada, Modula-2, Clu, object-oriented languages like C++, Eiffel, etc.
I heard two weeks ago at a conference in Boston that 50% of all security
problems reported to CERT (center charged with reporting security problems
and solutions) are due to one particular feature of one specific programming
language. Given the increasing interest in programs communicating over the
internet, one has to wonder why those interested in security still write
in that language.
Most languages today designed to support specific software development
methodology or philosophy. E.g., top-down design, object-based design,
encapsulation, information-hiding.
Languages influence the way people think about programming process.
Faculty always complain about BASIC hackers.
Minimum requirements for programming languages:
-
Universal - if can solve on computer then can program it in the language
-
Natural (expressive) - easy to express ideas.
-
Implementable
-
Efficient (for writing, compilation, or execution?)
-
Reliable - writeable (high-level), readable, ability to deal with
exceptional behavior)
-
Maintainable - Easy to make changes, decisions compartmentalized, clean
interfaces.
Alternative Programming Language Paradigms
Important to be aware of different programming language paradigms,
allow one to think about problems in different ways.
Partially driven by new architectures (or at least not constrained by
old). Procedural paradigm (C, Pascal, FORTRAN, Ada, Modula-2) is
closest to machine architecture.
Other paradigms:
-
functional - Popular in AI (LISP & Scheme) and theoretical
prog. lang. community.
Closest to theoretical models and mathematics.
- logic - Originally of interest in AI (though fading).
Also important as database query languages.
Now shifting to "constraint" languages.
Declarative, control implicit in search.
-
object-oriented - Latest fad. All major systems must be
"object-oriented" to be up-to-date. Important, but current languages have
some flaws.
- Based on discrete simulation, objects responsible for knowing what to
do.
-
Package state with appropriate operations.
History of Programming Languages
Machine language -> Assembly language -> High-level languages
Programmers: Single highly trained programmer -> large teams which
must cooperate
Early
- FORTRAN - Backus @ IBM, 1957, 18 person-years to write first compiler,
- Goals & contributions: numerical problems, very efficient
compiler, separate compilation
- Many revisions FORTRAN II, IV, 66, 77, 90
- ALGOL 60 - Committee, 1960
- Goals & contributions: numeric, block structure, recursion,
elegant, very influential
- Ancestor of ALGOL W, ALGOL 68, Pascal, Modula, Ada, etc.
- COBOL - Committee, 1960
- Goals & contributions: business data processing, records
- Several revisions
Early Schisms:
- LISP - McCarthy @ MIT, 1962, core is functional
- Goals & contributions: List processing and symbolic manipulation,
AI
- Scheme & Common LISP are modern descendents
- APL - Iverson @ Harvard, IBM, 1960 (for notation),
calculator language for array computations.
- SNOBOL 4 - Griswold @ Bell Labs, 1966 - string processing via pattern
matching
Consolidation
- PL/I - IBM committee, 1967
- combine FORTRAN, COBOL, ALGOL 60 - but not integrated, now considered
a failure.
- Multipurpose - include ptrs, records, exceptions, etc.
Next Leap Forward
-
ALGOL 68 - Committee, "orthogonal" elements, elegant but very hard to
understand
-
Simula 67 - precursor of object-oriented languages - designed for
simulation, coroutines
-
Pascal - Wirth, ETH, 1971 - designed only as a teaching language
-
Support structured programming, spare & elegant
-
Successful beyond expectations
Abstract Data Types
-
Clu, Mesa, Modula-2, Ada
-
Supports modules for encapsulation and information hiding
Other paradigms
-
Object-oriented: Smalltalk (1972), Eiffel, C++, Object Pascal, Java
-
Functional: Scheme (1985?), ML (1978), Miranda (1986) & Haskell
(1991)
-
Logic: PROLOG (1972), newer constraint programming languages.
4th generation languages
-
Important in business applications
-
Specialized packages of powerful commands w/simplified "user-friendly"
syntax.
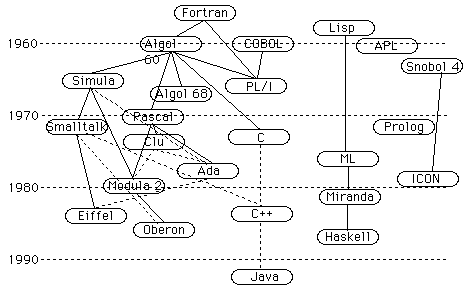
Topics to be covered in course:
-
Programming language features and organization, including modules,
classes,
exception handlers, generic types
-
functional and object-oriented, as well as procedural
paradigms. (No longer consider logic programming important enough to spend
time on.)
-
Programming language support for reliable programming:
abstraction, encapsulation, information hiding, polymorphism,
higher-order operators.
-
Language support for concurrency
-
Formal definitions of programming languages
-
Compilers and interpreters
-
Run-time behavior of programming languages, including impact of binding
time.
Three main concerns:
- Programming language features for reliable programming
- Run-time behavior of programming languages
- Formal semantics and interpreters for implementing languages.
Start out by learning ML so can explore some new ideas & rapidly
program interesting applications.
Write our own interpreters for simple languages so can see impact of
various design decisions.
Functional Languages
Problems with imperative (command-based) languages
In his 1978 Turing award lecture (granted in recognition of his role in
the development of FORTRAN, ALGOL 60, and BNF-grammars), John Backus
attacked the pernicious influence of imperative programming languages and
their dependence on the von Neumann architecture.
What is problem with imperative languages?
Designed around architectures available in 1950's.
Components:
-
CPU with accumulator and registers.
-
Memory
-
Tube connecting CPU with Memory which transmits one word at a time.
To execute an instruction, go through fetch, decode, execute cycle.
Ex. To execute statement stored in location 97 (ADD 162):
- Fetch instruction from memory location 97 (to CPU)
- Decode into operation (ADD) and address (162)
- Fetch contents of address.
- Add contents to accumulator and leave result in accumulator.
Simple statement like A:=B+C results in several accesses to memory
through "Von Neumann bottleneck."
Imperative program can be seen as control statements guiding execution
of a series of assignment statements (accesses and stores to memory).
Variable in programming language refers to location whose contents may
vary with time.
Hard to reason about variables whose values are always changing, even
within same procedure or function.
Math notation not like that. Static. If want to add time, add new
parameter. Gives static reasoning about dynamic processes.
Important notion called referential transparency. Can replace an
expression anywhere that it occurs by its value.
Very important for parallelism, since compute once and then reuse.
Not true of imperative languages. Can't compute x+1 once and replace
all occurrences by its value.
Order of execution in imperative programs very important - inhibits
parallel execution.
We will see several advantages of functional programming.
- Referentially transparent - easier to reason about, easier to make
parallel. Once expression evaluated, it can be reused.
- Order of execution need not be specified. Expressions can
automatically be executed when needed, even in parallel.
- Higher-level, resulting in shorter, more understandable programs.
- Can build new higher-order functions which allow you to put together
old programs in more flexible ways.
- "Lazy evaluation" can allow one to compute with infinite objects.
Other important reasons to study functional languages:
- Useful in AI research
- Useful in developing executable specifications and prototype
implementations.
- Closely related to CS theory (e.g., recursive functions, denotational
semantics).
Back to:
kim@cs.williams.edu